Redis是一种基于客户端-服务端模型以及请求/响应协议的TCP服务。这意味着通常情况下一个请求会遵循以下步骤:
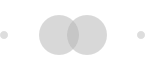
客户端向服务端发送一个查询请求,并监听Socket返回,通常是以阻塞模式,等待服务端响应。
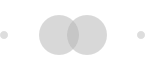
服务端处理命令,并将结果返回给客户端。

Redis 管道技术
Redis 管道技术可以在服务端未响应时,客户端可以继续向服务端发送请求,并最终一次性读取所有服务端的响应。
实例
查看 redis 管道,只需要启动 redis 实例并输入以下命令:
$(echo -en "PING\r\n SET runoobkey redis\r\nGET runoobkey\r\nINCR visitor\r\nINCR visitor\r\nINCR visitor\r\n"; sleep 10) | nc localhost 6379
+PONG
+OK
redis
:1
:2
:3
以上实例中我们通过使用 PING 命令查看redis服务是否可用, 之后我们设置了 runoobkey 的值为 redis,然后我们获取 runoobkey 的值并使得 visitor 自增 3 次。
在返回的结果中我们可以看到这些命令一次性向 redis 服务提交,并最终一次性读取所有服务端的响应
管道技术的优势
管道技术最显著的优势是提高了 redis 服务的性能。
一些案例和测试数据
案例一:基本使用
$redis = new Redis();
//开启管道模式
$pipe = $redis->multi(Redis::PIPELINE);
//循环遍历数据,执行操作
foreach ($users as $user_id => $username)
{
// 用户被访问的次数+1
$pipe->incr('accessed:' . $user_id);
// 获取用户数据记录
$pipe->get('user:' . $user_id);
}
// 开始执行管道里所有命令
$users = $pipe->exec();
// 打印数据
print_r($users);
案例二:将100万条数据写入redis
//产生100万条数据到指定文件
declare(strict_types=1);//开启强类型模式
function random($length, $numeric = false)
{
$seed = base_convert(md5(microtime() . $_SERVER['DOCUMENT_ROOT']), 16, $numeric ? 10 : 35);
$seed = $numeric ? (str_replace('0', '', $seed) . '012340567890') : ($seed . 'zZ' . strtoupper($seed));
if ($numeric) {
$hash = '';
} else {
$hash = chr(rand(1, 26) + rand(0, 1) * 32 + 64);
$length--;
}
$max = strlen($seed) - 1;
for ($i = 0; $i < $length; $i++) {
$hash .= $seed{mt_rand(0, $max)};
}
return $hash;
}
$filePath = './data.txt';
for ($i = 0; $i <= 1000000; $i++) {
$str = random(10, true);
file_put_contents($filePath, $str . PHP_EOL, FILE_APPEND);
}
//读取数据通过管道方式写入到redis
$lines = file_get_contents($filePath);//获取文件内容
ini_set('memory_limit', '-1');//不要限制Mem大小,否则会报错
$arr = explode(PHP_EOL, $lines);//转换成数组
//echo $arr['1000000'] ?? 'null';
try {
$redis = new \Redis();
$redis->connect('192.168.1.9', 6379);
$redis->auth('*****');//密码验证
$redis->select(0);//选择库
$redis->pipeline();//开启管道
foreach ($arr as $key => $value) {
$redis->hsetNx('helloworld', (string)$key, $value);
}
$redis->exec();
echo $redis->hGet('helloworld', '1000000') . PHP_EOL;
echo $redis->hGet('helloworld', '1000001') . PHP_EOL;
} catch (\Exception $e) {
echo $e->getMessage();
}
案例三:通过管道批量设置与读取
//批量设置
try {
$redis = new \Redis();
$redis->connect('192.168.1.9', 6379);
$redis->auth('******');
$redis->select(0);
$redis->pipeline();//开启管道
$redis->set('str1', 'h');
$redis->set('str2', 'e');
$redis->set('str3', 'l');
$redis->set('str4', 'l');
$redis->set('str5', 'o');
$redis->set('str6', 'w');
$redis->set('str7', 'o');
$redis->set('str8', 'r');
$redis->set('str9', 'l');
$redis->set('str10', 'd');
$result = $redis->exec();
print_r($result);
} catch (\Exception $e) {
echo $e->getMessage();
}
结果:
Array
(
[0] => 1
[1] => 1
[2] => 1
[3] => 1
[4] => 1
[5] => 1
[6] => 1
[7] => 1
[8] => 1
[9] => 1
)
//批量读取
try {
$redis = new \Redis();
$redis->connect('192.168.1.9', 6379);
$redis->auth('******');
$redis->select(0);
$redis->pipeline();//开启管道
$redis->get('str1');
$redis->get('str2');
$redis->get('str3');
$redis->get('str4');
$redis->get('str5');
$redis->get('str6');
$redis->get('str7');
$redis->get('str8');
$redis->get('str9');
$redis->get('str10');
$result = $redis->exec();
print_r($result);
} catch (\Exception $e) {
echo $e->getMessage();
}
结果:
Array
(
[0] => h
[1] => e
[2] => l
[3] => l
[4] => o
[5] => w
[6] => o
[7] => r
[8] => l
[9] => d
)